画像のビット数(8や24など)やチャンネル数(色の数、Lの場合は1、RGBの場合は3など)は画像処理をするときに、画像データを直接参照する場合などに必要になってきます。
jpegファイル(*.jpg)を開いたときには bits という値が拾え、1画素、1色あたり8bitであることがわかります。
しかしながら、他の形式のファイル(少なくとも bmp, pmg, gif)では、この bits の値がありません。
そこで、どのファイルでも画像のビット数やチャンネル数を調べられるようにするには、mode の値を調べるようにします。
modeの取得は、以下のように行います。
from PIL import Image
# Pillow で画像を読み込む
pil_image = Image.open("image_color.bmp")
# modeの表示
print("モード:", pil_image.mode)
モードの種類は以下の通りです。
mode | 説明 |
1 | 1-bit pixels, black and white, stored with one pixel per byte |
L | 8-bit pixels, black and white |
P | 8-bit pixels, mapped to any other mode using a color palette |
RGB | 3×8-bit pixels, true color |
RGBA | 4×8-bit pixels, true color with transparency mask |
CMYK | 4×8-bit pixels, color separation |
YCbCr | 3×8-bit pixels, color video format |
LAB | 3×8-bit pixels, the L*a*b color space |
HSV | 3×8-bit pixels, Hue, Saturation, Value color space |
I | 32-bit signed integer pixels |
F | 32-bit floating point pixels |
(参考)
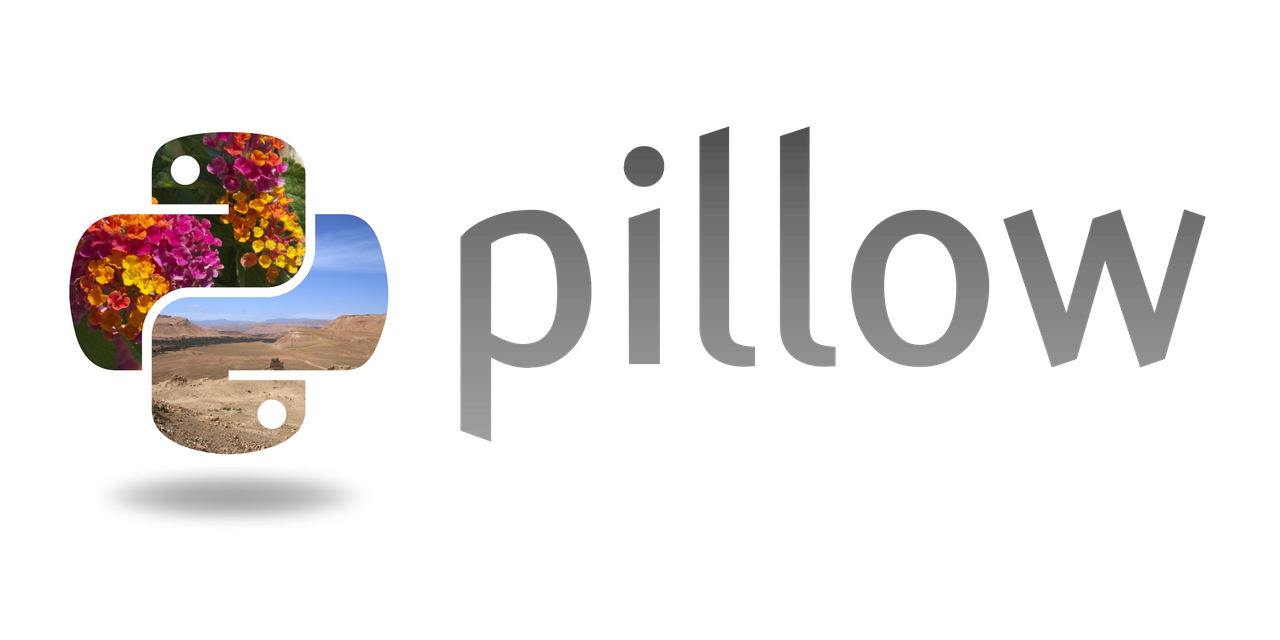
Concepts
The Python Imaging Library handles raster images; that is, rectangles of pixel data. Bands: An image can consist of one ...
画像のビット数やチャンネル数を調べるのに、この mode を取得して、条件分岐でビット数やチャンネル数を上記の表から取得すると正確に求まります。
ただ、実際に使われるのは L, RGB, RGBA の3つぐらいなので、1画素8bit限定として考えると、チャンネル数が求まればビット数も求まります。
チャンネル数を直接取得できる方法は無さそうなので、 getbands() というメソッドを使って行います。
この getbands() は、例えば mode = ‘RGB’ のとき、各チャンネルの色の名前の(‘R’, ‘G’, ‘B’)というタプルを返すメソッドになります。そのため、このタプルの長さを取得すればチャンネル数も求まります。
サンプルプログラム
from PIL import Image
# Pillow でモノクロ画像を読み込む
pil_image_mono = Image.open("image_mono.bmp")
print("■■ モノクロ画像 ■■")
print("モード:\t\t", pil_image_mono.mode)
print("バンド:\t\t", pil_image_mono.getbands())
print("チャンネル数:\t", len(pil_image_mono.getbands()))
# Pillow でカラー画像を読み込む
pil_image_color = Image.open("image_color.bmp")
print("■■ カラー画像 ■■")
print("モード:\t\t", pil_image_color.mode)
print("バンド:\t\t", pil_image_color.getbands())
print("チャンネル数:\t", len(pil_image_color.getbands()))
実行結果
参考
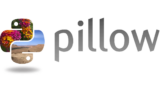
Image Module
The Image module provides a class with the same name which is used to represent a PIL image. The module also provides a ...
コメント